Table of Contents
You can easily convert Python code to Java. In finding a way to convert python code to Java, you come along with many choices but finding the best one that does not affect the code’s readability, and speed is essential.
Some recommend Tokenizer to complete it faster than you expect, or the Al Code translator also comes in handy for you. The work is more efficiently done using a utility, converter tools, or simple methods like String Replace Methods.
Al Code Translator
The Facebook research team developed the transcoder, which proved useful while converting programming languages. You can implement the transcoder in your local system.
Otherwise, you can implement it in the Google cloud. Google Cloud is an easier way to do it but doing it in a local system is also always available. By installing all the local dependencies, you can convert your content to Java code.
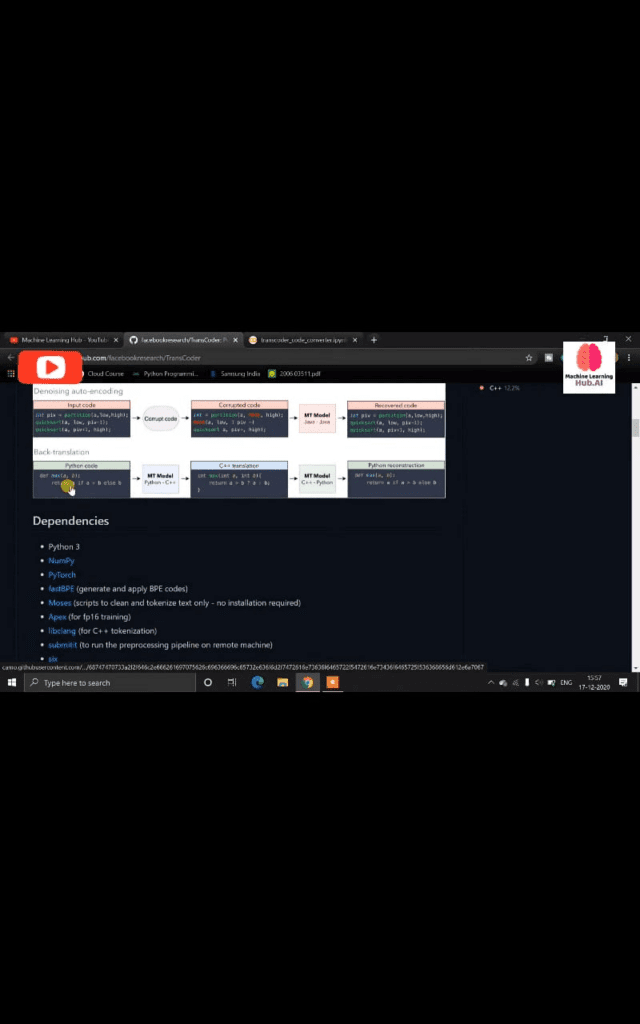
To implement Transcoder in the Google Cloud named Notebook, just follow the steps as guided.
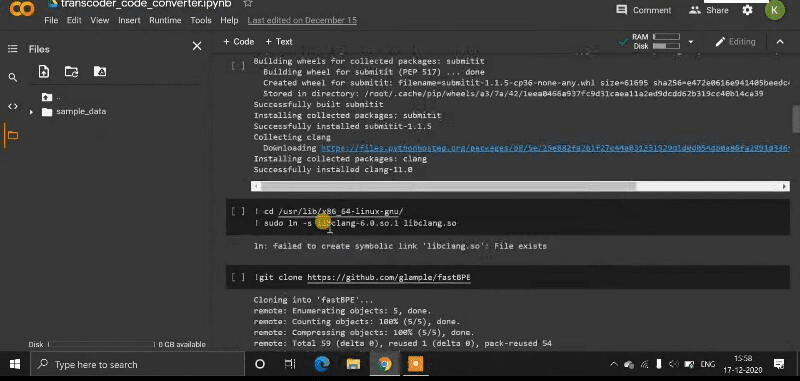
- Check the Python version you are using.
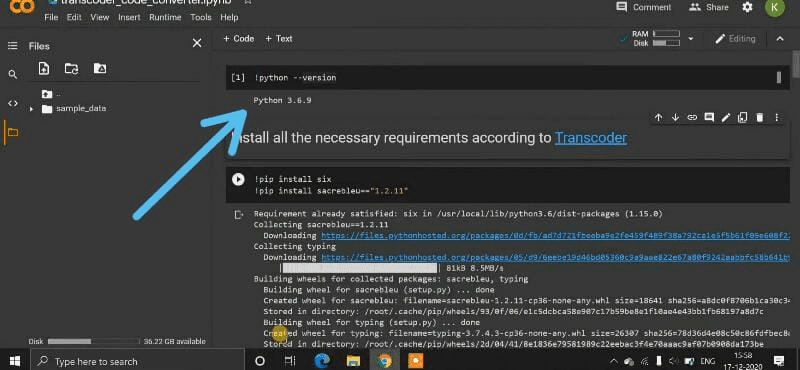
- According to the transcoder file, we have to install some requirements. So install them one by one.

It will show as,
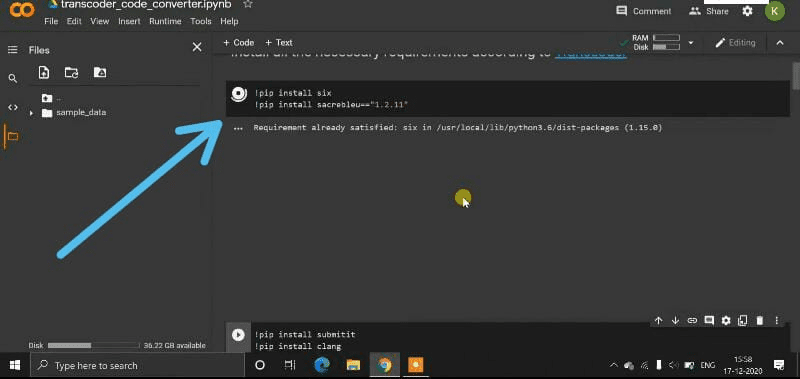
Similarly, it will ask you to download additional files like Linux files, supporting files, etc. you need to click and download them immediately. Just follow the steps, and it will be implemented.
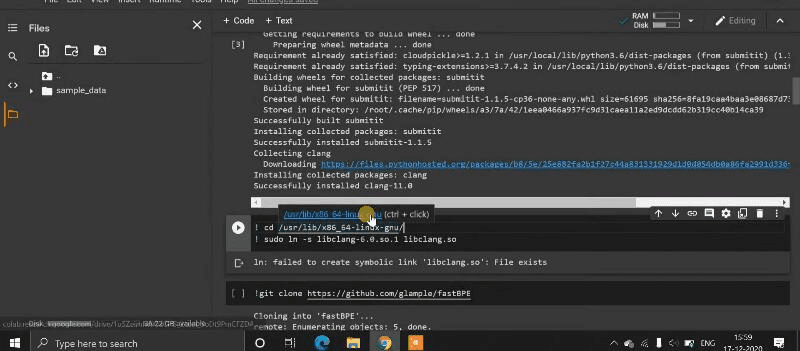
Using Tokenizer
One way is to use the String Replacement Method or the re Regular Expressions to get the exact result.
The following example can explain tokenization.
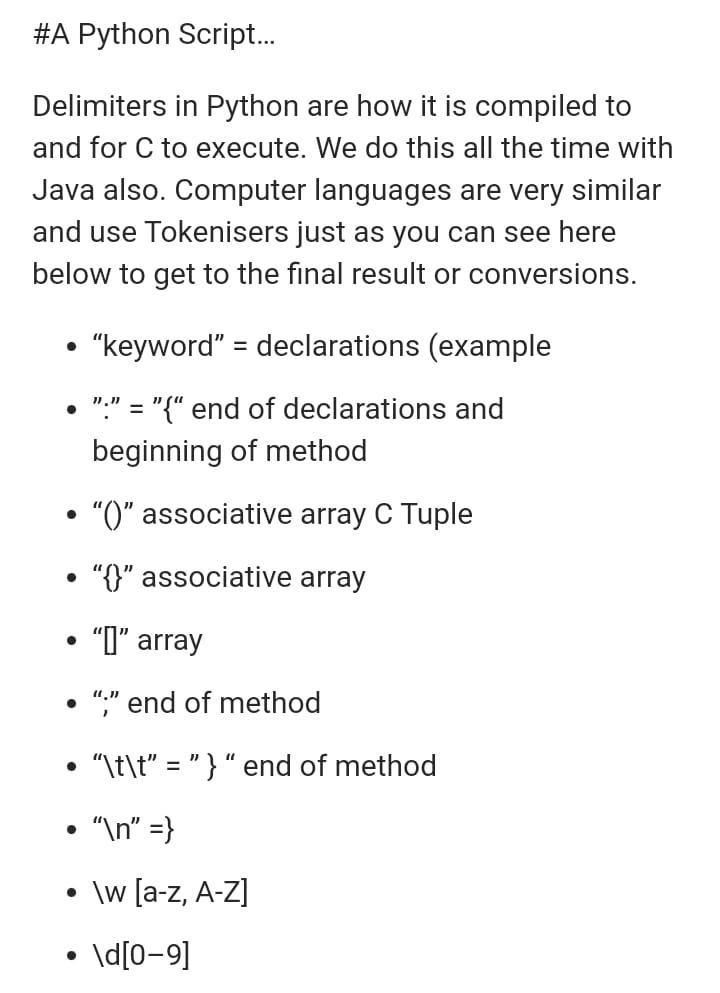

List of tools that can help you convert Python code to Java
You can also let your code written in Python run in Java by using the following application:
- Jython: Jython is an implementation of Python that is integrated with Java. It allows you to run Python code on the Java Virtual Machine (JVM) and access Java libraries from Python code. You can also use Jython to convert Python code to Java. Jython provides a tool called “jythonc” that can compile Python code into Java bytecode. However, Jython is not designed to convert arbitrary Python code to Java, and some features of Python may not be available in Jython.
- PyDev: PyDev is an open-source Python IDE for Eclipse that provides code completion, debugging, and other features for Python development. PyDev also includes a code converter that can convert Python code to Java. PyDev’s code converter is not perfect, and some manual adjustments may be required.
- Transcrypt: Transcrypt is a Python-to-JavaScript compiler that can also generate Java bytecode from Python code. Transcript is designed to provide a subset of Python features that can be compiled into efficient and readable JavaScript or Java code. Transcrypt includes a tool called “transcrypt” that can convert Python code to Java.
- Jpype: Jpype is a Python module that allows you to call Java code from Python and vice versa. Jpype can also be used to convert Python code to Java. However, Jpype is not a code converter per se, and it requires some manual adjustments to make the converted code work correctly.
- Javasnoop: Javasnoop is a tool that allows you to intercept and modify Java method calls at runtime. Javasnoop can also be used to convert Python code to Java. To use Javasnoop, you need to start a Java application and attach Javasnoop to it. Javasnoop will then intercept the Java method calls made by the application and display them in a GUI. You can then modify the intercepted calls to generate Java code that performs the same operations as the original Python code.
- Pyj2c: Pyj2c is a Python-to-C++ compiler that can also generate Java bytecode from Python code. Pyj2c is designed to provide a subset of Python features that can be compiled to efficient and readable C++ or Java code. Pyj2c includes a tool called “pyj2c” that can convert Python code to Java. However, Pyj2c is not perfect, and some manual adjustments may be required.
- JythonCodeAnalyzer: JythonCodeAnalyzer is a tool that can analyze Jython code and generate equivalent Java code. JythonCodeAnalyzer is designed to work with Jython code specifically, so it may not be suitable for converting arbitrary Python code to Java. However, if you have Jython code that you want to convert to Java, JythonCodeAnalyzer can be a useful tool.
- Javapy: Javapy is a Python-to-Java code converter that uses a combination of machine learning and natural language processing techniques to analyze Python code and generate equivalent Java code. Javapy is designed to work with a wide range of Python code and can handle complex Python code structures. However, Javapy is still under development, and its accuracy may vary.
What Are Some Subtle Differences Between Python and Java?
Python and Java despite being among the popular programming languages display some notable differences, whether in the form of their syntax or fundamentals. This is why it’s so important to learn about them so you can streamline your conversion process. These include:
1) Syntax Disparities
· Whitespace vs. Braces: In Python, it’s common to use indentations to specify code blocks, while Java relies more on curly brackets {}. This is one of the key things that is implemented in Python to Java converters available online.
· Dynamic vs Static Code: Python is based on dynamic code meaning it allows variables to change type. On the other hand, Java is more focused on static typing, requiring the on-spot declaration of variables. So, you must define the typed structure before starting the conversion.
· Simplicity vs Complexity: Python is generally praised in the coding community for its readability and concise syntax. It’s written in a relatively straightforward layout, and each line is interpreted beforehand so you can make changes if required. In contrast, Java is technically complex and statically typed, so you must deal with longer code segments.
2) Fundamental Distinction
· Compiled vs Interpreted: Python’s code works on interpretation so that there can be faster development and testing, whereas Java’s code complies first with bytecode. This can make things hard for those wanting to manually convert Python code to Java.
· Functional Programming: Functional programming is a key feature in Python, which means you can treat functions as first-class citizens. The approach greatly differs in Java so you may require additional adjustments during code conversion.
· Object-Oriented Approach: Both of these languages support object-oriented programming; however, their implementation can definitely vary. Java adheres to more strict OOP principles (interfaces and strict class structures), while Python behaves the opposite.
Converting a Sample Code from Python To Java – Manually
Here is a clear example of taking a simple program in Python and turning it into an equivalent program written in Java.
Consider the following Python function that calculates the factorial of a number:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
result = 1
for i in range(2, n + 1):
result *= i
return result
# Test the function
number = 5
print(f”The factorial of {number} is {factorial(number)}”)
STEPS
1) Analyze Code Structure
· First and foremost, it’s extremely important to understand the purpose and logic of the given Python function ( “factorial”).
· Similarly, you also need to check the conditional statements and loop used for factorial calculation.
2) Identify language-specific constructs
· Once that’s done, try to identify Python’s syntax elements such as “def”, “if-else”, “for loop”, and “range()” functions.
· Python also uses indentations to define code blocks, so you probably need to understand them before you convert code to Java.
3) Mapping Python Functionalities to Java Equivalents
· In certain cases, creating a Java class/method is necessary to calculate the end values (factorial).
Converted Python code in Java:
public class FactorialCalculator {
public static void main(String[] args) {
int number = 5;
System.out.println(“The factorial of ” + number + ” is ” + factorial(number));
}
// Method to calculate factorial
public static int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
int result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
}
}
· Explanation
ü Here, we’ve created a Java class termed “FactorialCalculator”.
ü We’ve also used a “main” method to test the “factorial” output.
ü Then Python’s “factorial” function is translated into a Java method.
ü As for Java syntax, we have changed the conditional statements (“if-else”) and a “for” loop to calculate the factorial.
This code can be compiled to run the “FactorialCalculator” class. However, you need to verify that it actually calculates the factorial correctly.
Any Alternative for Python to Java converter online?
Using 3rd party online converters can be a little bit problematic especially when you’re dealing with distinct programming languages such as Python and Java.
For once they lack consistency so you are going to waste quite a lot of time in debugging. Similarly, there is the issue with safety and privacy, so you aren’t sure what you are getting into.
One of the better ways to translate your code is through Bing’s new AI chatbot. It’s based on Open AI’s GPT4 model, which is surprisingly fast and accurate. Plus, it’s compatible with advanced languages such as C++ and Java, so you won’t have to look for anything else.
Microsoft’s Bing Chatbot
Here is a basic Python code that will be fed in the Bing AI:
NumberA = int(input(‘Enter First Number Here’))
NumberB = int(input(‘Enter Second Number Here’))
print(NumberA + NumberB)
It’s converted through a simple prompt “convert this Python code into Java code”:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print(“Enter First Number Here: “);
int NumberA = input.nextInt();
System.out.print(“Enter Second Number Here: “);
int NumberB = input.nextInt();
System.out.println(NumberA + NumberB);
}
}
As for testing, we are using an online compiler:
FAQs
What is the top free Python to Java converter online?
There are several third-party tools to convert your code to Java; however, the one that’s popular among programmers is Jython.
Can all Python code be converted directly to Java?
Unfortunately, not all Python code is interconvertible to Java, mainly due to fundamental differences between these languages.
What are a few things to understand for code conversion manually?
To convert your Python code into an advanced language such as Java, you need to understand things like syntax translation, data type, and variables and dependencies.
Wrap up